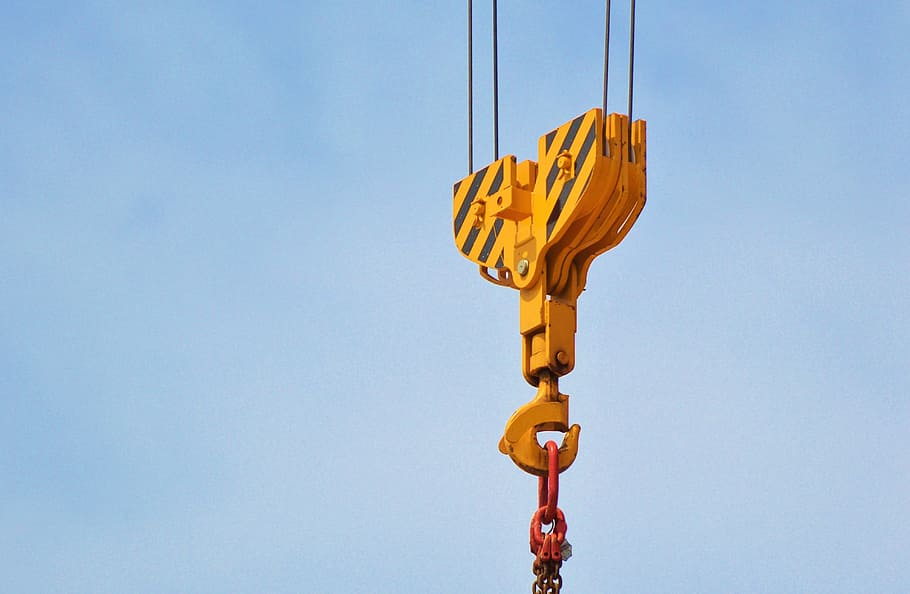
What is hoisting and why should you care?
Hoisting means you can do this
x = 5;
var x;
console.log(x) //5
plus5(5);
function plus5(a) {
console.log(a+" plus 5 is "+(a+5)); //5 plus 5 is 10
}
Hmm, looks okay doesn’t it? Hang on, the variable x
is being initialized before it is declared. Yes, that is because the interpreter has placed the declaration to the top of the file for you. You don’t see this happening it is just magic. The same goes for functions as well. Here the function plus5
is called before it is defined. Spooky.
Only declarations using ‘var’ are hoisted
var a = 5; //Initialize a
var result;
c=2; // Initialization causes declaration, no hoisting
//Display a and b and c, b at this point is undefined because it
//is initialized on the next line
result = a+" "+b+" "+c;
var b = 7; // Initialize b
console.log(result); // 5 undefined 2
In the example above b
is undefined when result
is initialized. This is because only the declaration is hoisted and not the assignment. This is the same as writing
var a,b; //Declare a and b
a=5; //Initialize a
var result; //Declare result
result=a+" "+b; //Display a and b,b is undefined at this point
b = 7; //Initialize b
console.log(result); //5 undefined
This behaviour can lead to unexpected behaviour in your code.
‘let’ and ‘const’ keywords are not hoisted
If you use these ES6 keywords then you do not need to be concerned about hoisting. If it doubt then ditch var
and use const
or let
instead.